Javascript Get Data Attribute
Are you j
But if you want a bit more information about using Javascript to get Data Attributes, then let’s get started.
HTML5 Data attributes are a really cool and useful way of storing more information in your HTML code and help extend the front-end functionality of a webpage or mobile app.
But how do you use Javascript to get the data attribute?
What is a Data Attribute?
Firstly, let’s define what I mean by Data Attribute to make sure we’re all on the same page.
Data Attributes are custom HTML attributes that can be added to any HTML5 item and allow us to store extra information we can use and manipulate.The data is localised to the web page or application they’re on and therefore can only be manipulated on that web page or application.
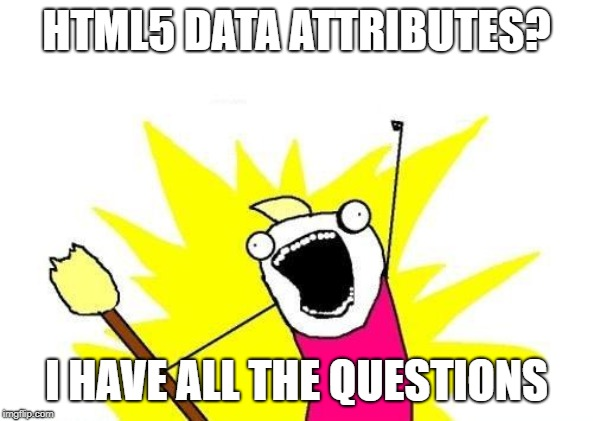
So what data can or should be stored using Data Attributes? What is the correct syntax to use? When should Data Attributes be used?
Let’s take a look at a couple of Data Attribute pointers / best practices directly from W3C to answer those questions:
- Data tags should begin with the data- prefix and must contain at least one character after the hyphen. For example, data-tag or data-nav etc.
- Custom Data Attributes should only really be used to store custom data and are not intended to replace existing HTML attributes and elements.
- Data Attributes will work on any HTML element.
- Each HTML element can have any number of Data Attribute properties.
- Data Attributes can have any value.
A Real-World Example of Data Attrbutes
Let’s take a look at a real-world example of the HTML5 Data Attribute syntax and what you might use it for:
<a id='link-1'
data-link='1'
data-target='1'
data-product-title='Worship Coffee T-Shirt'
data-product-img='product.jpg'
data-product-link='https://www.thewebdeveloperguide.com'
class="link'>
Worship Coffee Tee</a>
In the example above, we have a regular HTML anchor tag. It has a standard HTML ID and Class, but as you can see it has a number of HTML5 Data Attributes too.
Some of these Data Attributes are out of context and are meaningless at this point, but others are a little more obvious.
In our real-world example we’re going to be making a simple item picker using these HTML5 Data Attributes and Javascript. We’re going to create links and a content area and the idea is we’re going to use the data we store in the above anchor tag’s Data Attributes to build our display.
Let’s take a look at the Data Attributes in a little more detail:
- A data-link attribute to help us identify the link from the others (we’ll be adding more links later).
- A data-target attribute, which we’re going to use to work out where we’re going to build our display.
- A data-product-title attribute, which is going to contain the name of our product.
- A data-product-
img attribute which is going to contain the location of the image we want to display. - A data-product-link attribute which is going to contain a link to where the user can buy the product.
Now we’ve stored our data using Data Attributes, let’s take a look at how to access and use it.
How Can I Use Javascript to Get a Data Attribute?
There are a couple of different ways to use Javascript to get a Data Attribute:
- Using the .dataset property.
- Using the .getAttribute property.
Okay, so what’s the difference between the two? The answer is: not much. Obviously, the syntax is a little different for each property but they can be used to basically do the same thing: extract data.
The main difference is that the dataset property is solely for accessing custom data in Data Attribute, whereas the getAttribute property is to get data from any attribute within an HTML element.
Interestingly, each of the methods have slightly different performance results. Using the .dataset to get all of the dataset attributes was a little faster than using .getAttribute, HOWEVER, using getAttribute to access a single Data Attribute was actually faster.
Also, both properties are pretty much fully supported:
In terms of which property is better to use, I think it pretty much comes down to personal preference so let’s take a look at both methods in action.
Using Javascript Get to a Data Attribute using Dataset
The first thing we need to do is to grab the HTML element so we can access it’s properties. There are a couple of ways you can do this using Vanilla Javascript:
- Get the element by its id using the Javascript document.getElementById function.
- Or grab the element by a dataset itself using the Javascript document.querySelector function.
Let’s take a look at that in the code.
First, here’s a little reminder of the HTML element we want to access.
<a id='link-1'
data-link='1'
data-target='1'
data-product-title='Example Product'
data-product-img='product.jpg'
data-product-link='https://www.thewebdeveloperguide.com'
class="link'>
Worship Coffee Tee</a>
Here’s how we get the element by its ID using the Javascript document.getElementById function:
const link = document.getElementById('link-1');
And here’s how we get the same element by a Data Attribute using the Javascript document.querySelector function:
const link = document.querySelector('[data-link="1"]');
In the above example, I used the data-link Data Attribute as the hook to grab the element in Javascript because that’s what I intended the function of that Data Attribute to be. However, I could’ve grabbed the element using any of the Data Attributes in that element with Javascript document.querySelector – include the class attribute.
Now that we have the HTML element, let’s access a couple of our Data Attribute’s using the Javascript Dataset property.
link.dataset.target
// This will get us 1
link.dataset.productTitle
// This will get us 'Example Product'
link.dataset.productImg
// This will get us 'product.jpg'
Did you notice the dataset syntax in both of those examples? The prefix of data- is removed and the attribute name remains. For the second Data Attribute the dash is removed and converted to a camelCase format.
This is because the functionality uses DOMStringMap to handle key transformations.
Using Javascript to Get a Data Attribute using getAttribute
Now let’s get our custom data from the Data Attribute using the getAttribute Javascript function.
We still have to get the element using either the document.getElementById or document.querySelector functions like before, but we access the data like this:
const link = document.getElementById('link-1');
Or like this:
const link = document.querySelector('[data-link="1"]');
So now we get the Data Attributes by:
link.getAttribute('data-target');
// This will get us 1
link.getAttribute('data-product-title');
// This will get us 'Example Product'
link.getAttribute('data-product-img');
// This will get us 'product.jpg'
Again, you’ll notice the syntax used to grab the Data Attribute is completely different from using the dataset function. When using the getAttribute function, you have to use the full Data Attribute term to access its content.
This is because the getAttribute function is able to access all the attributes in the element (like the class element for example), not just Data Attributes.
Bonus: Access Data Attributes using CSS
While we’re on the subject of Data Attributes, it’s also worth mentioning that you can access and style them directly using CSS. Here’s a really basic example:
See the Pen Styling Data Attributes Directly by Kris Barton (@TheWebDeveloperGuide) on CodePen.
Okay, so this functionality may have limited use in real-world coding but it’s certainly a cool little bit of functionality worth knowing.
Using Javascript to get Data Attributes: A Real-World Example
Before I finish this article, it’s worth exploring how a web developer may want to use Data Attributes in a real-world example.
Here’s the scenario: we want a simple HTML/CSS/Javascript item picker (or maybe tab) component to allow users to choose from a series of cool t-shirt designs.
We load all of the relevant data on page load and store it all in Data Attributes within the component’s relevant HTML elements.
We’ll be storing:
- The product title.
- The product image URL.
- The product link.
For the User Interface, we’re going to display the product title’s as clickable links, with
The first product will be displayed on page load and the idea is for the user to click the product links to display the relevant product and information.
Let’s take a look at the Codepen:
See the Pen HTML5 Data Attributes – Read World Example – dataset by Kris Barton (@TheWebDeveloperGuide) on CodePen.
Further Reading
- https://www.w3.org/TR/2011/WD-html5-20110525/elements.html#embedding-custom-non-visible-data-with-the-data-attributes
- https://developer.mozilla.org/en-US/docs/Learn/HTML/Howto/Use_data_attributes
- https://www.w3schools.com/tags/att_global_data.asp
- https://webdesign.tutsplus.com/tutorials/all-you-need-to-know-about-the-html5-data-attribute–webdesign-9642
- https://www.sitepoint.com/how-why-use-html5-custom-data-attributes/
- https://developer.mozilla.org/en-US/docs/Web/API/HTMLElement/dataset
- https://www.w3schools.com/jsref/met_element_getattribute.asp
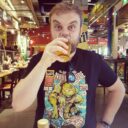